Append String in Python – How can we do it?
Strings are textual immutable data type in Python. The major purpose of string is to link multiple strings into one new object. It is an elementary string operation when merging two or more strings into one.
Concatenation is another word, which is used when considering append string in Python. Therefore, append and concatenate are used interchangeably.
Here, there will be joining or adding the value of one string to another string.
What are the Methods to append string in Python?
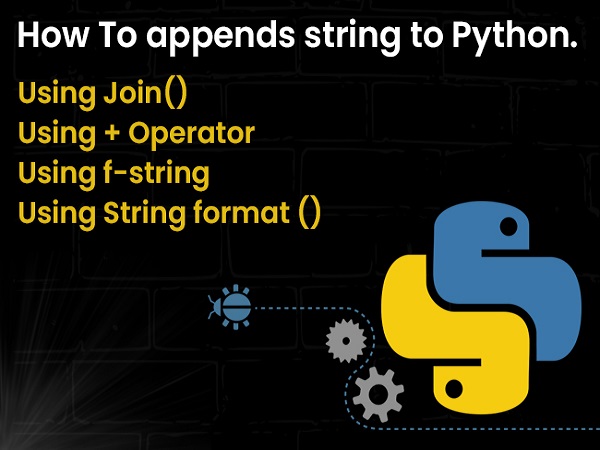
Method 1: Using join ()
The join () method concatenates iterable objects like tuples, list or dictionaries into one string. Following code is useful in call join () on a string.
String.join(<iterable object>)
The string acts like a separator between the joined object.
Following example show the join () method in use
Input
first_name ="Hello"
second_name = "World"
print("first_name:", first_ name)
print("second_name:", second_name)
list = [first_name, second_name]
string_txt = "".join(list)
print("appended string", string)
Output
The first name: Hello
The second name: World
The appended string: HelloWorld
Here we are calling the join() method on the string with a space and provide a list of strings appends the strings. Between each element, there will be a separator. The string 1 is Hey and string 2 is world. Therefore, joint string and final output will be Hey World.
Method 2 Using + Operator
This is another method to append string to python. Here, the plus (+) operator join the two strings into one object. The syntax will be as follow.
<string 1> + <string 2>
The strings are either variables that store string types or string literals.
first_name ="Hello"
second_name ="World"
print("first_name:", first_name)
print("second_name:", second_name)
first_name += second_name
print("appended string:", first_name)
Output
The first name: Hello
The second name: World
The appended string: HelloWorld. All you need to use + operator to join the strings and make an output. This operator is slow for appending more than two strings. Here also, the final output will be Hey World.
Method 3: Using f-string
The Python f-string is a new way to format the strings. They are less prone to errors and more readable. The f-string method use literal string interpolation to format stings.
first_name = "Hello"
second_name = "World"
print("first name:", first_name)
print("second name", second_name)
string = f"{first_name}{second_name}"
print("The appended string:", string)
Output
The first name: Hello
The second name: World
The appended string: HelloWorld
Method 4: Using String format ()
Heading tags helps in distinguishing the main section and subsections of the page. This is how those sections are related to each other.
Using curly brackets ({}) to define the placeholder and passing the values into the format () method.
“{} {}”.format (,)
The provided values in the format() method are then inserted into placeholder spaces.
str1 = “Hey”
str2 = “World”
print (“{} {}”.format (str1, str2))
The format() method allowing the controlling the string format and also appending order.
str1 = "Hey"
str2 = "World"
print("{0} {1}".format (str1, str2))
Output
Hey World
Verdict:
You can use any method for append string in Python. The use of += operator is better for few strings. On the other hand, the use of join{} function is useful in appending multiple strings. Hope you got familiar with all the different methods of appending a string in Python. Any Python developer should know the string appending in Python.