How Can We Integrate Zoom in React js?
Zoom is a common video conferencing platform for professionals. No doubt, it is easy, but the zoom OAuth app is another merit for the professionals. In this we integrate zoom in react js for the convenience of user. It allows users to create, update or remove zoom meetings from your portal itself. Business professionals can create an online meeting and then share it with others.
In this article we will explain you about the zoom integration. We will also learn how to create Zoom OAuth app.
- Initially you need to create an OAuth app by following guide on Zoom App Marketplace.
- Make a note of Client ID, Client Secret and Redirect URL.
- We will cover meeting: read, meeting: write and user: read
The simple and fast approach makes it possible to get on a project on time. It is the prime factor for companies to include Vuejs development services.
Note: It is important to verify your Zoom app, otherwise only your account will work.
How to integrate zoom in react-native?
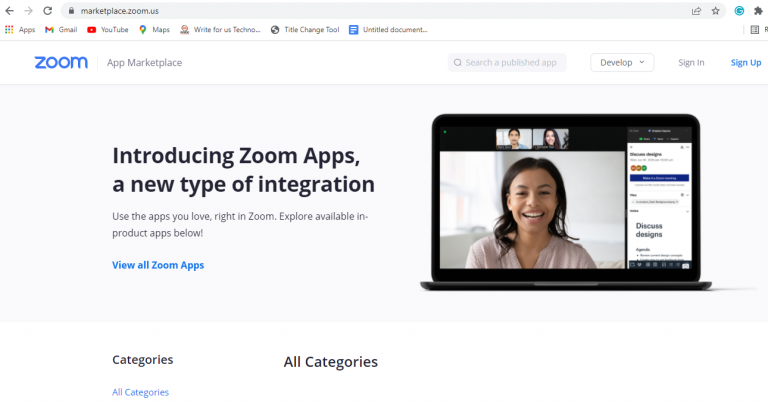
Setup OAuth integration:
Firstly connect a zoom link on your portal. Set up env variables like ZOOM_API_KEY and ZOOM_REDIRECT_URL.
<a href={`https://zoom.us/oauth/authorize?
response_type=code&client_id=${process.env.ZOOM_API_KEY}&redirect_uri=${process.env.ZOOM_REDIRECT_URL}`}>
Connect Zoom
</a>
Now retrieve code query parameter and pass it to your server. Also, you can use React useEffect for this purpose.
React.useEffect(() => {
if (router.query.code) {
fetch (‘/connectZoom’, {
method: ‘POST’,
headers: {
‘Content-Type’: ‘application/json’,
},
body: JSON.stringify ({ code: router.query.code }),
}).then(() => {
/* Show success message to user */
}).catch(() => {
/* Show error message to user */
});
}
}, [router.query.code]);
Further make a request to zoom with code and complete integration on the server.
try {
const b = Buffer.from(process.env.ZOOM_API_KEY + “:” + process.env.ZOOM_API_SECRET);
const zoomRes = await fetch(`https://zoom.us/oauth/token?
grant_type=authorization_code&code=${req.body.code}&redirect_uri=${process.env.ZOOM_REDIRECT_URL}`, {
method: “POST”,
headers: {
Authorization: `Basic ${b.toString(“base64”)}`,
},
});
if(!zoomRes.ok)
return res.status(401).send(“Could not connect with Zoom”);
const zoomData = await zoomRes.json();
if (zoomData.error)
return res.status(401).send(“Could not connect with Zoom”);
// Retreive user details
const zoomUserRes = await fetch(“https://api.zoom.us/v2/users/me”,
{
method: “GET”,
headers: {
Authorization: `Bearer ${zoomData.access_token}`,
},
});
const zoomUserData = await zoomUserRes.json();
/*
Encrypt and store below details to your database:
zoomUserData.email
zoomUserData.account_id
zoomData.access_token
zoomData.refresh_token
zoomData.expires_in // convert it to time by adding these seconds to current time
*/
return res.send({
/* Return necessary data to frontend */
});
} catch (e) {
return res.status (500) .send (“Something went wrong”) ;
}
Now you can use stored data to make requests to zoom.
Refresh Token
It is better to compare the stored access token expiry time with the current time before making any request. Use the refresh token, if the access token has expired. Using following codes to obtain a new access token.
try {
const b = Buffer.from(process.env.ZOOM_API_KEY + “:” + process.env.ZOOM_API_SECRET);
const zoomRes = await fetch(`https://zoom.us/oauth/token?
grant_type=refresh_token&refresh_token=${req.body.refresh_token}`, {
method: “POST”,
headers: {
Authorization: `Basic ${b.toString(“base64”)}`,
},
});
if(!zoomRes.ok)
return res.status(401).send(“Could not connect with Zoom”);
const zoomData = await zoomRes.json();
if (zoomData.error)
return res.status(401).send(“Could not connect with Zoom”);
/*
Encrypt and update below details on your database:
zoomData.access_token
zoomData.refresh_token
zoomData.expires_in // convert it to time by adding these seconds to current time
*/
/* Return necessary data */}
catch (e) {
return res.status(500).send(“Something went wrong”);
}
Create a meeting:
Firstly make a post request to the user meetings endpoint using stored user zoom email. Access the token to create a new meeting. Check API docs for the request and response properties. Follow the below command to create a meeting in zoom integration.
fetch(`https://api.zoom.us/v2/users/${data.email}/meetings`, {
method: “POST”,
headers: {
Authorization: `Bearer ${data.accessToken}`,
“Content-Type”: “application/json”,
},
body: JSON.stringify({
/*
Pass necessary details like
start_time in UTC
topic
agenda
duration
*/
}),
});
Store the returned meeting details and then show on UI as per the need.
Update the meeting:
Now Make a Patch request to meeting id. API Ref.
fetch(`https://api.zoom.us/v2/meetings/${meetingId}`, {
method: “PATCH”,
headers: {
Authorization: `Bearer ${accessToken}`,
“Content-Type”: “application/json”,
},
body: JSON.stringify({
/*
Pass details that needs to be updated
*/
}),
});
Delete meeting:
To delete meeting just make a Delete Request to the meeting id. API ref.
fetch(`https://api.zoom.us/v2/meetings/${meetingId}`, {
method: “DELETE”,
headers: {
Authorization: `Bearer ${accessToken}`,
“Content-Type”: “application/json”,
},
});
Deauthorization Endpoint:
To get the Zoom OAuth app verified you need to provide the deauthorization endpoint which will call Zoom Data Compliance API. In the zoom integration you need to verify the Zoom OAuth app.
try {
const b = Buffer.from(process.env.ZOOM_API_KEY + “:” + process.env.ZOOM_API_SECRET);
/* Use req.body.payload.account_id, compare it with stored Zoom account, find and clear user Zoom related details
*/
// Call Zoom Compliance API
await fetch(`https://api.zoom.us/oauth/data/compliance`, {
method: “POST”,
headers: {
Authorization: `Basic ${b.toString(“base64”)}`,
“Content-Type”: “application/json”,
},
body: JSON.stringify({
client_id: req.body.payload.client_id,
user_id: req.body.payload.user_id,
account_id: req.body.payload.account_id,
deauthorization_event_received: req.body.payload,
compliance_completed: true,
}),
});
} catch (e) {
console.log(“Zoom deauthorize event error “, e);
}
Wrapping up!
This was all about React zoom integration to make the zoom meeting easy. If you are a React js developer then you can do it easily. We hope you get the knowledgeable words through this blog. Share your comments to show off your thoughts.