Know Everything About React 18 Which is Available on npm:
In our last blog, we discussed the expected features of React 18. That time the React 18 Release date was not final. Now finally it has been officially made available on npm. This new version includes many handy features like automatic batching, streaming server-side rendering and startTransition.
Concurrent React is also a part. However, it is only available when you use a concurrent feature. Here we will unlock every crucial information about React 18.
What is Concurrent React?
Instead of a feature, you can refer to concurrency as the mechanism that enables React to prepare multiple versions of the UI. React uses sophisticated processes in its internal implementation. The react concurrent mode might be a bit complicated for the library maintainer.
React uses sophisticated techniques in the internal implementation. However, you will not see these concepts anywhere in the public APIs.
Top React 18 New Features:
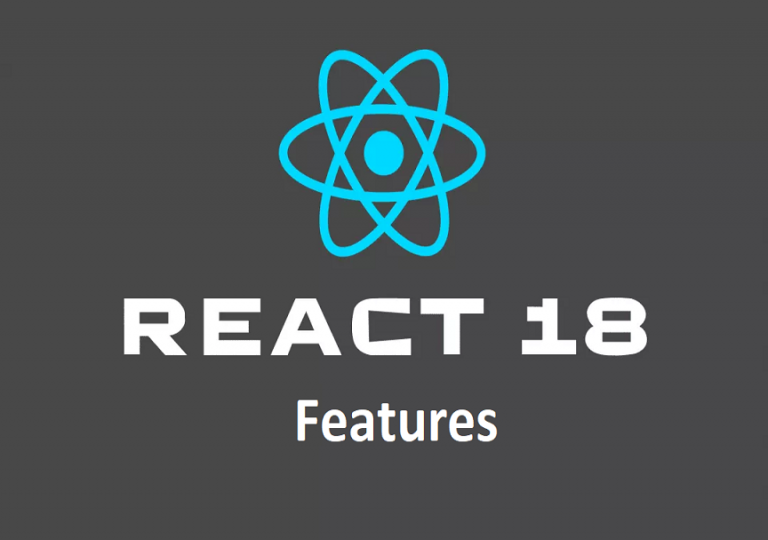
Automatic Batching:
Batching begins when React groups multiple state updates into a single re-render for better performance. We only batched updates inside React event handlers without automatic batching. Now, these updates will be automatically batched with automatic batching.
// Before: only React events were batched.
setTimeout(() => {
setCount(c => c + 1);
setFlag(f => !f);
// React will render twice, once for each state update (no batching)
}, 1000);
// After: updates inside of timeouts, promises,
// native event handlers or any other event are batched.
setTimeout(() => {
setCount(c => c + 1);
setFlag(f => !f);
// React will only re-render once at the end (that's batching!)
}, 1000);
New Feature: Transitions:
A new concept known as a transition in React will distinguish between urgent and non-urgent updates.
- Urgent updates reflect typing, pressing and clicking.
- Transition updates transition UI from one view to another
Urgent updates like clicking and typing need immediate response to match intuitions about how physical objects behave. The user doesn’t hope to see the intermediate value on the screen. That’s why transitions are different.
import {startTransition} from 'react';
// Urgent: Show what was typed
setInputValue(input);
// Mark any state updates inside as transitions
startTransition(() => {
// Transition: Show the results
setSearchQuery(input);
});
All the updates wrapped in startTransition are treated as non-urgent. If more urgent updates like key presses or clicks come in.
- useTransition: It is a hook to start transitions.
- StartTransition: It is a procedure to start transitions when the hook is not in use.
The startTransition API will keep your app responsive during the large screen updates also. It means your app will no longer be unresponsive during the heavy update operations.
New suspense SSR and Architectural improvements:
React 18 has introduced architectural improvements to the react server side rendering. The server side rendering produces HTML from React components on the server. This is how clients can now see the page content before the JavaScript bundle load.
However, there is a drawback of SSR. You must have your data ready for components on the server. This happens because It does not allow components to wait for the data. This issue can be overcome by two methods- selective hyderation of the client and Streaming HTML.
Selective Hyderation of the client:
Using selective hydration, components will not block hydration. It will start hydrating without blocking another component.
Streaming HTML on the server:
Using suspense, the react will send the statistical pieces of the UI component. It will decide which component part will take longer time to load and what can be directly rendered. It benefits by cutting down the waiting time for the user to see the initial UI render.
New Suspense Features:
The suspense will specify the loading state for a part of the component tree.
<suspense fallback={<Spinner/>}>
<Comments/>
</Suspense>
Suspense develops the “UI loading state”, a first-class declarative concept in the React programming model.
In React 18, there is the integration of support for Suspense on the server. It also works best when mixed with transition API. React will show the render until enough data has loaded to prevent the bad loading state.
New Client and Server Rendering APIs:
Now we took the opportunity to redesign the APIs that we expose for rendering on the server and client. These changes enable users to continue using the old APIs in React 17 mode.
React DOM Client:
The following new APIs are now exported from react-dom/client:
createRoot: This is a new method to create a root to render or unmount. You can use it instead of ReactDOM.render.
hydrateRoot: It is a new way to hydrate a server-rendered app. Professionals can use it instead of ReactDOM.hydrate.
Both of them accept the new option called onRecoverableError in case you want to be notified. React will use reportError or the console.error by default.
React DOM Server:
These latest APIs will be now exported from react-dom/server. It also supports streaming suspense on the server.
renderToReadableStream: It is for the modern edge runtime environment. The typical examples are Deno and Cloudflare workers. The current renderToString process keeps working.
New Strict Mode Behaviors:
They will add new features that will enable React to add and remove sections of the UI. For instance, React must show the previous screen immediately when the user tabs away from the screen.
React will mount the component and also create the effects:
*React mounts the component.
*Layout effects are created.
*Effects are created
React will simulate unmounting and remounting components in the development mode.
*React mounts the components.
* Layout effects are created.
* Effects are created.
*React simulates unmounting the component.
*Layout effects are destroyed
*Effects are destroyed
*React simulates mounting the component with previous state
*Layout effects are created
*Effects are created
New Hooks:
useId:
It is a new hook for creating unique IDs on both client and server. Meanwhile, it will avoid hydration mismatches. It is also useful for the component libraries integrating with accessibility APIs.
useTransition:
useTransition and startTransition will enable you to mark some state updates as not urgent. By default, the other state updates are considered urgent. React will allow urgent state updates to interrupt non-urgent state updates.
useDeferredValue:
This hook will let you defer the re-rendering of a non-urgent part. It is identical to the debouncing but contains many merits.
useSyncExternalStore:
It is a new hook through which external stores support concurrent reads by forcing updates to the store to be synchronous. It eliminates the need for useEffect while implementing subscriptions to external data sources.
Verdict:
React 18 is out, and it contains many new features. It will benefit developers and the overall project outcome. For now, we can say that it will accelerate the project’s workflow. It will help professionals to speed up their work. Ultimately, the results will also be truly beneficial.